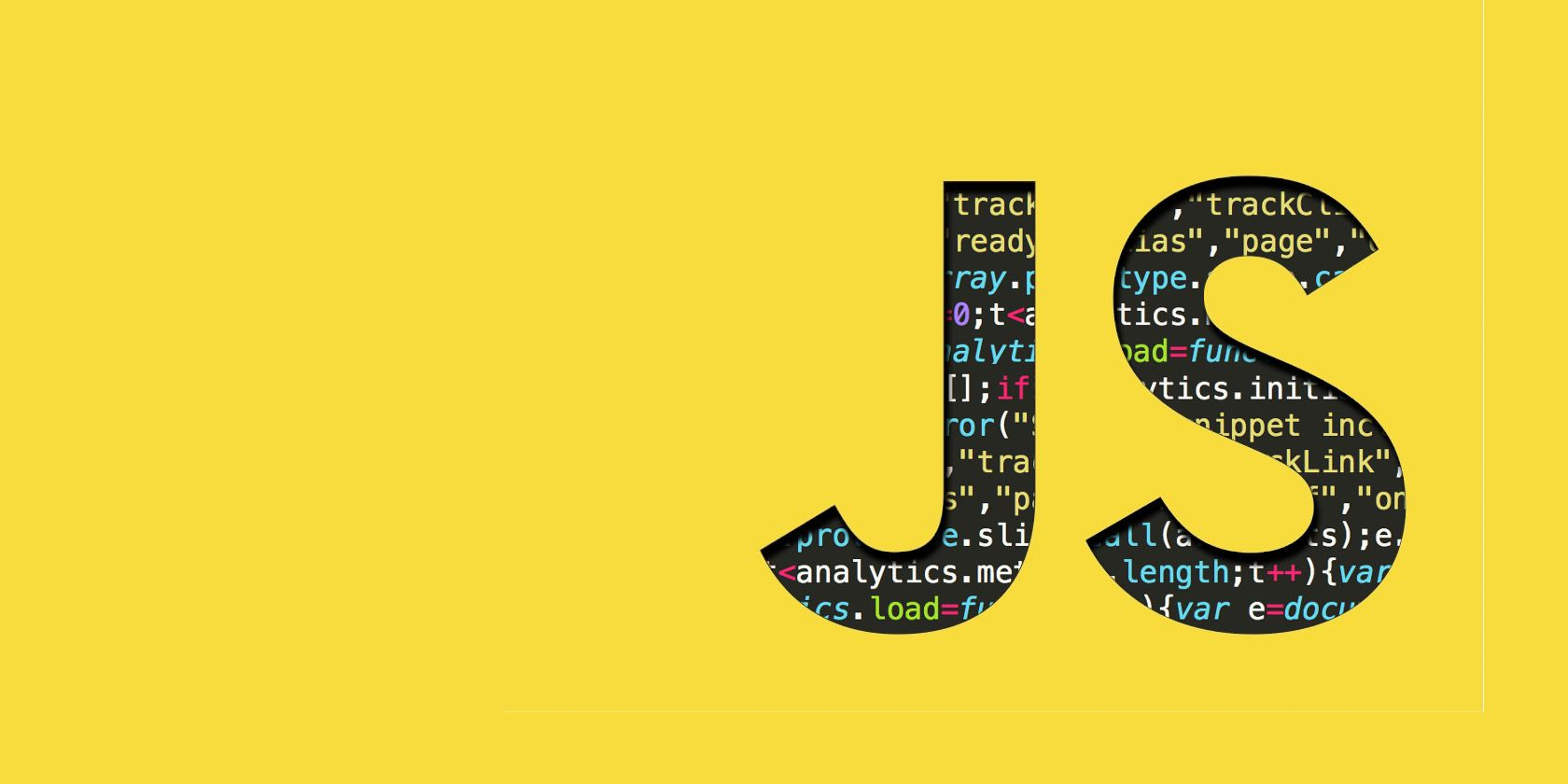
1 min read
JavaScript: Get array with unique objects
In JavaScript you can use Set
to get an array with unique items. However, this does not work with objects. Converting the object to a string before creating the set is the solution.
Here is an example:
// A list that contains a duplicate object
list = [
{
source: "A",
target: "B",
},
{
source: "B",
target: "C",
},
{
source: "A",
target: "B",
},
];
// Generate set with stringified list objects and then parse to array of objects
unique = [...new Set(list.map((o) => JSON.stringify(o)))].map((s) =>
JSON.parse(s)
);
// In result there is unique list
console.log(unique);
// [ { source: 'A', target: 'B' }, { source: 'B', target: 'C' } ]
Tags: array , filter , javascript
Improve this page
Show statistic for this page