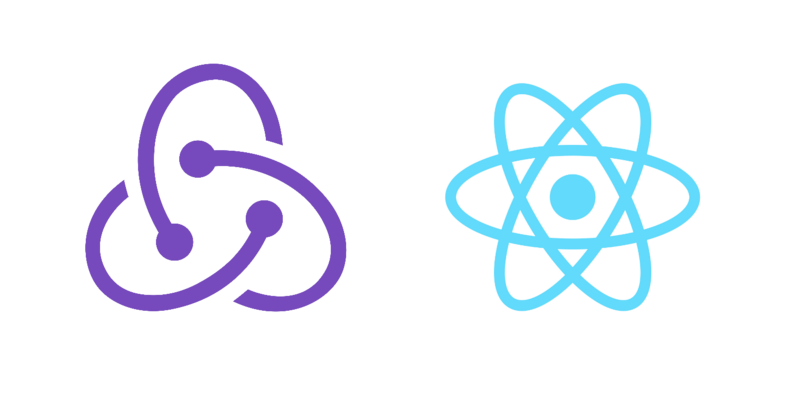
1 min read
Debounce a redux dispatch method in a react component
Tightly connected reactivity in a react application has the side effect that it is sometimes necessary to delay the execution of a method. Assume you have a search input field that filters elements while typing, every field input creates a search request. In order to get rid of unnecessary search requests you have to wait until a user has finished typing and then start the search. To make this work without a search button, you have to intercept repeating executions (debounce) of the search method within a specified time frame and delay the execution of the last call.
Here’s a simple example of a react search component where the redux dispatch method will be debounced.
import React from 'react'
import { Card, CardText, TextField } from 'material-ui'
import { setUserFilter } from '../actions'
import { debounce } from 'lodash'
class UserSearch extends React.Component {
constructor(props){
super(props)
this.updateFilter = debounce(this.updateFilter, 500)
}
updateFilter(){
let { dispatch } = this.props
let { filter } = this.refs
dispatch(setUserFilter(filter.getValue()))
}
render() {
return <Card>
<CardText>
<TextField
ref="filter"
onChange={this.updateFilter.bind(this)} />
...
</CardText>
</Card>
}
}
The search is only dispatched if the last call of the update filter method is older than 500ms.
Source: Stack Overflow - Perform debounce in React.js
Categories: JavaScript developmentTags: react , redux , search
Improve this page
Show statistic for this page