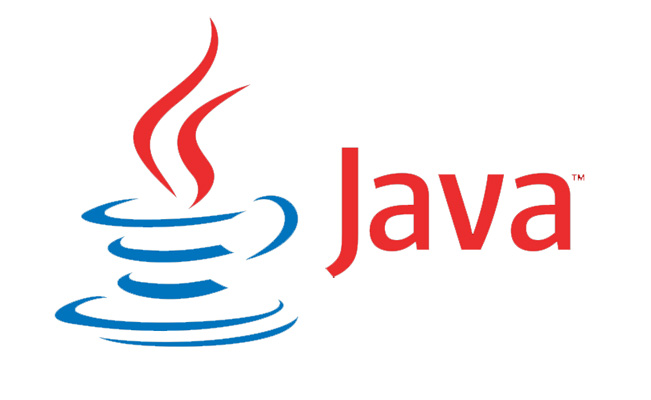
4 min read
Build a Java 3-tier application from scratch - Part 2: Model setup
Last time we’ve set up our basic project structure with gradle. This time we are going to create the models aka our Java classes. Below is uml diagram showing all classes, interfaces and their relationship.
Common Eclipse
Open the common project in eclipse and update it according to the instructions.
- Create a project structure like this:
- Update the class and interface files with the content showed below.
DAO.java
package ch.issueman.common;
import java.io.Serializable;
import java.util.List;
public interface DAO<T, Id extends Serializable> {
public void persist(T t);
public T getById(Id id);
public List<T> getAll();
public void update(T t);
public void delete(T t);
public void deleteAll();
}
We will use this interface later on for our controllers. It simply defines the allowed methods to communicate between the layers.
Model
package ch.issueman.common;
public interface Model {
public int getId();
}
No models without the models interface. For a DRY(don’t repeat yourself) code approach we will use polymorphism and a generic controller.
Person.java
package ch.issueman.common;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import lombok.Data;
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
@Entity
@Data
@Inheritance(strategy=InheritanceType.JOINED)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Person implements Model{
@Id
@GeneratedValue
private int id;
private String name;
public Person(){}
public Person(String name) {
super();
this.name = name;
}
}
This is the parent class for person related objects.
@Entity
: This is a java persistence table@Data
: By providing this annotations lombok creates getter and setter methods for this class.@Inheritance(strategy=InheritanceType.JOINED)
: Use the JOINED strategy for child classes.@JsonIgnoreProperties(ignoreUnknown = true)
: Ignore unknown properties when mapping json data to POJO.Id
: This field is the primary key.@GeneratedValue
: It will be generated by the DBMS.
User.java
package ch.issueman.common;
import javax.persistence.Entity;
import lombok.Data;
@Entity
@Data
@EqualsAndHashCode(callSuper=false)
public class User extends Person {
private String email;
private String password;
private String role;
public User(){}
public User(String name, String email, String password, String role) {
super(name);
this.email = email;
this.password = password;
this.role = role;
}
}
This is a child class of the Person class. We will use this class to authenticate the client against the webservice later.
@EqualsAndHashCode(callSuper=false)
this annotation is required whenever you’re extending from a class.
Employer.java
package ch.issueman.common;
import javax.persistence.Entity;
import lombok.Data;
@Entity
@Data
@EqualsAndHashCode(callSuper=false)
public class Employer extends Person {
private String company;
public Employer(){}
public Employer(String name, String company) {
super(name);
this.company = company;
}
}
Another child class of the Person class. Every project is owned by an employer.
Comment.java
package ch.issueman.common;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Lob;
import javax.persistence.ManyToOne;
import lombok.Data;
@Entity
@Data
public class Comment implements Model{
@Id
@GeneratedValue
private int id;
@Lob
private String comment;
@ManyToOne
private User user;
public Comment(){}
public Comment(String comment, User user) {
super();
this.comment = comment;
this.user = user;
}
}
A project can have multiple commands written by a user.
@Lob
: Create a large object field in the database for this attribute.@ManyToOne
: multiple foreign entities of type user can point to an entity of type comment.
Project.java
package ch.issueman.common;
import java.util.List;
import javax.persistence.CascadeType;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.ManyToMany;
import javax.persistence.ManyToOne;
import lombok.Data;
@Entity
@Data
public class Project implements Model {
@Id
@GeneratedValue
private int id;
private String title;
@ManyToOne
private Employer employer;
@ManyToMany(cascade = CascadeType.ALL)
private List<Comment> comments;
public Project() {
}
public Project(String title, Employer employer) {
super();
this.title = title;
this.employer = employer;
}
}
A project contains multiple comments and is owned by an employer.
@ManyToMany(cascade=CascadeType.ALL)
: It this entity is deleted, persisted, update apply all events to this foreign entity.
Here’s a list of all cascade types:
- CascadeType.PERSIST : means that save() or persist() operations cascade to related entities.
- CascadeType.MERGE : means that related entities are merged into managed state when the owning entity is merged.
- CascadeType.REFRESH : does the same thing for the refresh() operation.
- CascadeType.REMOVE : removes all related entities association with this setting when the owning entity is deleted.
- CascadeType.DETACH : detaches all related entities if a manual detach occurs.
- CascadeType.ALL : is shorthand for all of the above cascade operations.
- That was it. If you got this far you’ve successfully created the required models for our project.
Update
- 2015-04-01: Added @Data annotation from lombok to all models.
- 2014-04-03: List of all cascade types added.
Links
- Part 1: Introduction and project setup
- Part 2: Model setup
- Part 3: Object-relational mapping
- Part 4: Webservice
- Part 5: Client controller
- Part 6: Client view
Source
Feedback from Reddit on this post
Categories: Software developmentTags: three tier , application , eclipse , java
Edit this page
Show statistic for this page