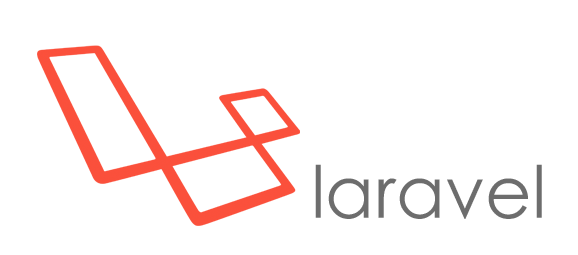
2 min read
Let’s write a Laravel application - Form validation for your backend and frontend
Form validation is always part of your data process workflow. With Laravel you can to validate the input on the controller or the eloquent model. To provide a seamless user experience it’s better to validate a form with JavaScript in the frontend. However due to security reasons you have provide a backend validation, otherwise it would possible to inject non valid data.
Luckily Bilal Gültekin came up with this awesome solution.
The ideas is simply avoid writing validation rules twice (backend and frontend) and rather reuse the backend validation rules by adding them as parameter to our frontend form. The extension requires the jquery validation plugin.
Let’s get started by installing the required assets.
Install the Laravel extension with composer.
composer.json
"require": {
"bllim/laravel-to-jquery-validation": "*"
}
Register the new service provider.
app/config/app.php
'providers' => array(
'Bllim\LaravelToJqueryValidation\LaravelToJqueryValidationServiceProvider',
)
The extension comes with a configuration file and two assets. You have to publish them first.
php artisan config:publish bllim/laravel-to-jquery-validation
php artisan asset:publish bllim/laravel-to-jquery-validation
In the folder public/packages/bllim/laravel-to-jquery-validation/ you’ll find now two JavaScript files. Include them in your form view. You can also use bower to maintain the jquery validation plugin. In addition you have to add this piece of JavaScript to enable the frontend validation for your form.
$('form').validate();
Now that we are ready let’s add the validation rules to our model.
app/model/Member.php
class Member extends Eloquent{
public static $rules = [
'firstname' => 'required',
'lastname' => 'required',
'email' => 'required|email'
];
}
As I’ve said we have to validate the input data in backend anyway, so here’s an example of the store function.
app/controllers/MemberController.php
class MemberController extends \BaseController {
public function store()
{
$validator = Validator::make($data = Input::all(), Member::$rules);
if ($validator->fails()) {
return Redirect::back()->withErrors($validator)->withInput(Input::except('password'));
}
$member->create($data);
Session::flash('message', 'Successfully created Member!');
return Redirect::to('members');
}
]
In your form view you can now set the validation rules from your model.
{{Form::setValidation(Member::$rules);}}
Here’s an example of an compatible form definition for this kind of validation.
{{ Form::open(array('url' => 'members')) }}
<div class="form-group">
{{ Form::label('firstname', 'Firstname') }}
{{ Form::text('firstname', Input::old('firstname'), array('class' => 'form-control')) }}
</div>
<div class="form-group">
{{ Form::label('lastname', 'Lastname') }}
{{ Form::text('lastname', Input::old('lastname'), array('class' => 'form-control')) }}
</div>
<div class="form-group">
{{ Form::label('email', 'E-Mail') }}
{{ Form::text('email', Input::old('email'), array('class' => 'form-control')) }}
</div>
{{ Form::submit('Save', array('class' => 'btn btn-primary')) }}
{{ Form::close() }}
From now on the input of your form will be validated in the front- and backend with the same rules.
Categories: Web developmentTags: backend , jquery , laravel
Edit this page
Show statistic for this page